README
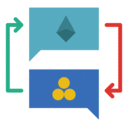
@acheetahk/algorithm
@acheetahk/algorithm 🧮 . Node.js common algorithm methods.
Please use version greater than 2.0.0
Methods Nav
Crypto
Installation
npm install @acheetahk/algorithm
cnpm install @acheetahk/algorithm
yarn add @acheetahk/algorithm
Dependencies
{
"@types/crypto-js": "^4.0.1",
"crypto-js": "^4.0.0"
}
Usage
import { Crypto } from '@acheetahk/algorithm';
hashing
Crypto.hashing('test') // 098f6bcd4621d373cade4e832627b4f6
Crypto.hashing('test', 'MD5') // 098f6bcd4621d373cade4e832627b4f6
hashing - args
param | type | require |
---|---|---|
data |
any |
true |
type |
MD5 (the default) SHA1 SHA256 SHA512 |
false |
encode
const result = Crypto.encodeStringify('test'); // 'dGVzdA=='
Crypto.encodeParse(result); // test
encodeStringify - args
param | type | require |
---|---|---|
data |
any |
true |
type |
Base64 (the default) Latin1 Hex Utf8 Utf16 Utf16LE |
false |
encodeParse - args
param | type | require |
---|---|---|
str |
string |
true |
type |
Base64 (the default) Latin1 Hex Utf8 Utf16 Utf16LE |
false |
simple aes
// supports AES-128, AES-192, and AES-256. It will pick the variant by the size of the key you pass in. If you use a passphrase, then it will generate a 256-bit key.
const result = Crypto.aesSimpleEncrypt('test', 'secret'); // auto aes-156 string
Crypto.aesSimpleDecrypt(result, 'secret') // test
aesSimpleEncrypt - args
param | type | require |
---|---|---|
data |
any |
true |
secret |
string |
true |
aesSimpleDecrypt - args
param | type | require |
---|---|---|
str |
string |
true |
secret |
string |
true |
aes
const result = Crypto.aesEncrypt('test', 'secret'); // ECE7672D1D7A33443AEDB29F3DCA6248
Crypto.aesDecrypt(result, 'secret') // test
aesEncrypt - args
param | type | require |
---|---|---|
data |
any |
true |
secret |
string |
true |
ivLength |
number (the default is 16 ) |
false |
modeType |
CBC (the default) CFB CTR CTRGladman OFB ECB |
false |
padType |
Pkcs7 (the default) Pkcs7 AnsiX923 Iso10126 Iso97971 ZeroPadding NoPadding |
false |
aesDecrypt - args
param | type | require |
---|---|---|
str |
string |
true |
secret |
string |
true |
ivLength |
number (the default is 16 ) |
false |
modeType |
CBC (the default) CFB CTR CTRGladman OFB ECB |
false |
padType |
Pkcs7 (the default) Pkcs7 AnsiX923 Iso10126 Iso97971 ZeroPadding NoPadding |
false |