README
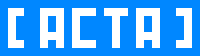
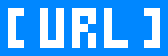
URL @acta/url
To parse URLs to formated objects and build URLs from objects.
Table of Contents
Parsing URLs
You can parse an URL that way:
import URL from '@acta/url';
const parsedURL = URL.parse('http://username:password@subdomain.domain.tld:8080/foo/bar/baz?key1=value1&key2=value2#hashvalue')
/**
{
auth: { password: 'password', username: 'username' },
domain: 'domain',
domainChain: ['tld', 'domain', 'subdomain'],
hash: 'hashvalue',
host: 'subdomain.domain.tld:8080',
hostname: 'subdomain.domain.tld',
href: 'http://username:password@subdomain.domain.tld:8080/foo/bar/baz?key1=value1&key2=value2#hashvalue',
isValidURL: true,
origin: 'http://subdomain.domain.tld:8080',
path: '/foo/bar/baz?key1=value1&key2=value2',
pathname: '/foo/bar/baz',
port: '8080',
protocol: 'http:',
query: 'key1=value1&key2=value2',
queryParams: { key1: 'value1', key2: 'value2' },
search: '?key1=value1&key2=value2',
tld: 'tld',
}
*/
The query parser is integrated.
When an element is missing, its value is null
(ex without hash: hash: null
).
Formating URLs
You can format an URL that way:
import URL from '@acta/url';
const formatedURL = URL.format({
auth: { password: 'password', username: 'username' },
domain: 'domain',
domainChain: ['tld', 'domain', 'subdomain'],
hash: 'hashvalue',
host: 'subdomain.domain.tld:8080',
hostname: 'subdomain.domain.tld',
href: 'http://username:password@subdomain.domain.tld:8080/foo/bar/baz?key1=value1&key2=value2#hashvalue',
isValidURL: true,
origin: 'http://subdomain.domain.tld:8080',
path: '/foo/bar/baz?key1=value1&key2=value2',
pathname: '/foo/bar/baz',
port: '8080',
protocol: 'http:',
query: 'key1=value1&key2=value2',
queryParams: { key1: 'value1', key2: 'value2' },
search: '?key1=value1&key2=value2',
tld: 'tld',
})
/**
* http://username:password@subdomain.domain.tld:8080/foo/bar/baz?key1=value1&key2=value2#hashvalue
*/
Dev scripts
npm run dev
to dev (build, test and watch)npm run build
to build the production versionnpm run release
to release a new version. Relies on release-it for deployments.
To develop in local using the package from another application or package, go for a symlink: npm link /the/absolute/path/url
.
To publish: npm publish --access=public
.