README
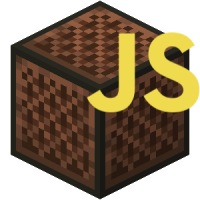
NBS.js
OpenNBS files, heavily inspired by NBSEditor and NoteBlockAPI.
A versatile API for reading, manipulating, and writing🔧 Including
🌐 Browser
It's recommended to use a versioned link, e.g.
@encode42/nbs.js@2.1.0
Script
<script src="https://cdn.jsdelivr.net/npm/@encode42/nbs.js"></script>
Minified: https://cdn.jsdelivr.net/npm/@encode42/nbs.js/dist/umd.min.js
Module
import { Song } from "https://cdn.jsdelivr.net/npm/@encode42/nbs.js/dist/esm.js";
Minified: https://cdn.jsdelivr.net/npm/@encode42/nbs.js/dist/esm.min.js
⚙️ Deno
import { Song } from "https://cdn.jsdelivr.net/npm/@encode42/nbs.js/dist/esm.js";
⚙️ Node.js
NPM
npm i @encode42/nbs.js
Yarn
yarn add @encode42/nbs.js
❔ FAQ
How do I use this?
Install NBS.js for your platform, then refer to the documentation and examples below.
Browser (Script)
<input type="file" id="file-input">
<script src="https://cdn.jsdelivr.net/npm/@encode42/nbs.js"></script> <!-- Import NBS.js -->
<script>
window.addEventListener("load", () => {
const input = document.getElementById("file-input");
// Clear the file input (QOL)
input.value = null;
// Initialize file input
input.addEventListener("change", () => {
const songFile = input.files[0]; // Read a NBS file
songFile.arrayBuffer().then(buffer => { // Create an ArrayBuffer
const song = NBSjs.fromArrayBuffer(buffer); // Parse song from ArrayBuffer
console.log(song);
});
});
});
</script>
Browser (Module)
index.html
<input type="file" id="file-input">
<script src="index.js" type="module">
index.js
import { fromArrayBuffer } from "https://cdn.jsdelivr.net/npm/@encode42/nbs.js/dist/esm.js"
window.addEventListener("load", () => {
const input = document.getElementById("file-input");
// Clear the file input (QOL)
input.value = null;
// Initialize file input
input.addEventListener("change", () => {
const songFile = input.files[0]; // Read a NBS file
songFile.arrayBuffer().then(buffer => { // Create an ArrayBuffer
const song = fromArrayBuffer(buffer); // Parse song from ArrayBuffer
console.log(song);
});
});
});
Deno
import { fromArrayBuffer } from "https://cdn.jsdelivr.net/npm/@encode42/nbs.js/dist/esm.js";
const songFile = await Deno.readFile("song.nbs"); // Read a NBS file
const buffer = new Uint8Array(songFile).buffer; // Create an ArrayBuffer
const song = fromArrayBuffer(buffer); // Parse song from ArrayBuffer
console.log(song);
Node.js
const fs = require("fs");
const { fromArrayBuffer } = require("@encode42/nbs.js"); // Import NBS.js
const songFile = fs.readFileSync("song.nbs"); // Read a NBS file
const buffer = new Uint8Array(songFile).buffer; // Create an ArrayBuffer
const song = fromArrayBuffer(buffer); // Parse song from ArrayBuffer
console.log(song);
Is there a demo?
Yes! A GitHub pages site is located here. It contains a demonstration of how to read and process NBS files, displays the song structure, and plays the song through the browser.
The demo is currently under development. Check NBSPlayer for a working example!
🔨 Building
Ensure Yarn and Node.js are installed.
- Enter the directory containing the NBS.js source code in your terminal.
- Install the build dependencies via
yarn install
. - Run
yarn run build
to generate the Node.js and browser modules.
Generated files:
dist/cjs.js
: CommonJS bundle, used by Node.js.dist/esm.js
: ES module for browser script modules.dist/umd.js
: UMD bundle for browser scripts.dist/*.min.js
: Minified bundle.build/
: Built ESNext JS files from TS.