README
iMatch.js
A vanilla JavaScript mobile-friendly before/after comparison slider, to view the difference between two images with mouse drag and touch swipe events.
Some Fetaures
- Both Desktop & Mobile
- Built-in Animation
- Touch Support
- Easy to Customise CSS Style
- Mobile-friendly
- Easy to intergrate
- Both Horizontal & Vertical Slide
- Pure JavaScript
PREVIEW
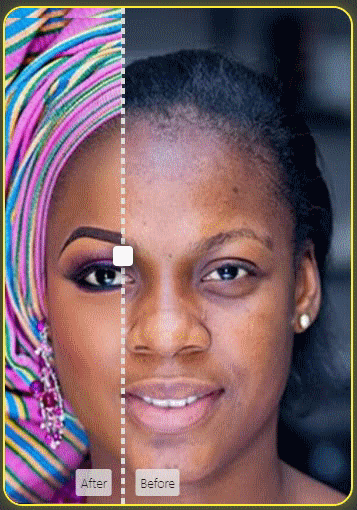
check index.html in samplePage folder for live Preview.
Installation
# Basic Node.JS installation
npm install @indaneey/imatch --save
Syntax
imatch()
from imatch
Import import { imatch } from '@indaneey/imatch';
Examples
require()
Calling iMatch using var iMatch = require('imatch');
var slider = new iMatch();
script tag
Calling iMatch using <head>
<script src="imatch.min.js"></script>
</head>
and then in script tag
create iMatch() instance
var slider = new iMatch();
Create Container With HTML Elements
First step, you have to create a container where you want your comparison slider to be. And add two different images inside with <img>
tags.
NOTE: Don't add three HTML Elements inside your container, because it will not works on three images, only two images are allowed. Also single one image will not work. JUST TWO(2) IMAGES!!
Example
<div class="container">
<img src="./assets/match1_1.jpg" alt="image One">
<img src="./assets/match1_2.jpg" alt="image Two">
</div>
Set Data Attribute To Container
To create a caption for your slider you have to set data attribute to your image tags.
Example
<div class="container">
<img data-caption="Before" src="./assets/match1_1.jpg" alt="image One">
<img data-caption="After" src="./assets/match1_2.jpg" alt="image Two">
</div>
The data attribute must be data-caption
then follow by your caption text.
Set Container Size
Here is most important part. Set your container width and height using CSS. And then iMatch.js will use these properties to create your slider.
NOTE: If not set width and height properties, the iMatch will not generate comparison slider for us.
Example
.container{
width: 300px;
height: 600px;
}
Attach Container To iMatch.js
After creating a container, you have to also create an iMatch instance too. And then attach them together.
Write your container class
attribute or id
attribute name as iMatch()
parameter in string format
Example
var slider = new iMatch('.container')
compare()
iMatch.js has a method called compare()
that will use to configure slider properties and display slider to screen.
Example
var slider = new iMatch('.container')
slider.compare()
NOTE: If not write this method, the iMatch.js will not generate comparison slider for us.
This method has different properties
- direction
- animation
- lineClassName
- arrowClassName
Example
var slider = new iMatch('.container')
slider.compare({
direction: 'HORIZONTAL', // horizontal or vertical value required in string
animation: true, // Boolean
lineClassName: 'line', // slider line class name for CSS style in string
arrowClassName: 'dot' // center rectangle class name for CSS style in string
})
direction property [ string ]
This property is used to set your slider movement direction between horizontal or vertical in string format.
animation property [ boolean ]
This property is used to enable or unable the build-in animation.
lineClassName property [ string ]
This property is a class name of slider line, it will be use to styling using CSS.
Example
var slider = new iMatch('.container')
slider.compare({
lineClassName: 'line', // slider line class name for CSS style in string
})
CSS
.line{
background-color: red
}
arrowClassName property [ string]
This property is a class name of center rectangle of line, it will be use to styling using CSS.
Example
var slider = new iMatch('.container')
slider.compare({
arrowClassName: 'dot', // slider line class name for CSS style in string
})
CSS
.dot{
background-color: red;
border-radius: 50%
}
iMatch.js
Developed by Indaneey_design. for any support contact me here