README
π§£re-promise
Retry failed api calls with an exponential back off timeπ Installation
yarn add @viveknayyar/re-promise
or
npm i @viveknayyar/re-promise --save
π§ Usage
// some js function in your code which fetches users data
const fetchUser = id => {
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve({ id: 'vivek', name: 'vivek' });
}, 10);
});
};
// with es6 await
import { retryPromise } from '@viveknayyar/re-promise';
async function retryAndFetchUser(id) {
try {
// await on this promise to resolve if you are using es6 await
const resp = await retryPromise({
fn: () => fetchUser(id),
retries: 3,
retryDelay: 2000,
retryOn: function(e) {
return [500, 502].includes(e.response.status);
}
});
} catch (e) {
console.log(e);
return Promise.reject(e);
}
}
// with traditional promises
import { retryPromise } from '@viveknayyar/re-promise';
function retryAndFetchUsers(id) {
return retryPromise({
fn: () => fetchUser(id),
retries: 3,
retryDelay: 2000,
retryOn: function(e) {
return [500, 502].includes(e.response.status);
}
}).then(resp => {
return resp;
}).catch(e => {
console.log(e);
return Promise.reject(e);
});
}
π§© Api
Props | Type | Default | Description |
---|---|---|---|
fn | function | null | function to call which should return a promise |
retries | number | 3 | The maximum amount of times to retry the operation. |
retryDelay | number | 3000 | The number of milliseconds before starting the retry. |
retryOn | function | null | function to specify a custom logic to decide if retry should be done or not |
backOffFactor | function | 1 | The exponential factor to use. Default is 1 |
debug | boolean | false | print information around retry attempts |
fn (required)
This function will be called every time we want to retry a promise or an api call. Make sure you return a promise from this function. It has the following signature:
function fn() {
return axios.get('some url');
}
retryOn (not compulsory)
This function will be called every time we have to decide if the promise should be retried or not. This function will be called with the error object so you can put in logic inside this function to return true or false based on which we will decide if the api call should be retried or not.
function retryOn(error) {
// logic to retry on certain status codes
return [502,500].includes(error.response.status);
}
backOffFactor (not compulsory)
This param will decide the exponential delay between retry attempts. For example:
// with es6 await and backOffFactor
import { retryPromise } from '@viveknayyar/re-promise';
async function retryAndFetchUser(id) {
try {
// await on this promise to resolve if you are using es6 await
const resp = await retryPromise({
fn: () => fetchUser(id),
retries: 3,
retryDelay: 2000,
backOffFactor: 3,
retryOn: function(e) {
return [500, 502].includes(e.response.status);
}
});
} catch (e) {
console.log(e);
return Promise.reject(e);
}
}
In the above case, our first retry call will happen after the retryDelay
of 2000ms.
The next retry call will now happen after retryDelay*backOffFactor
which is 6000ms and similarly we will continue for the next call.
π§³ Size
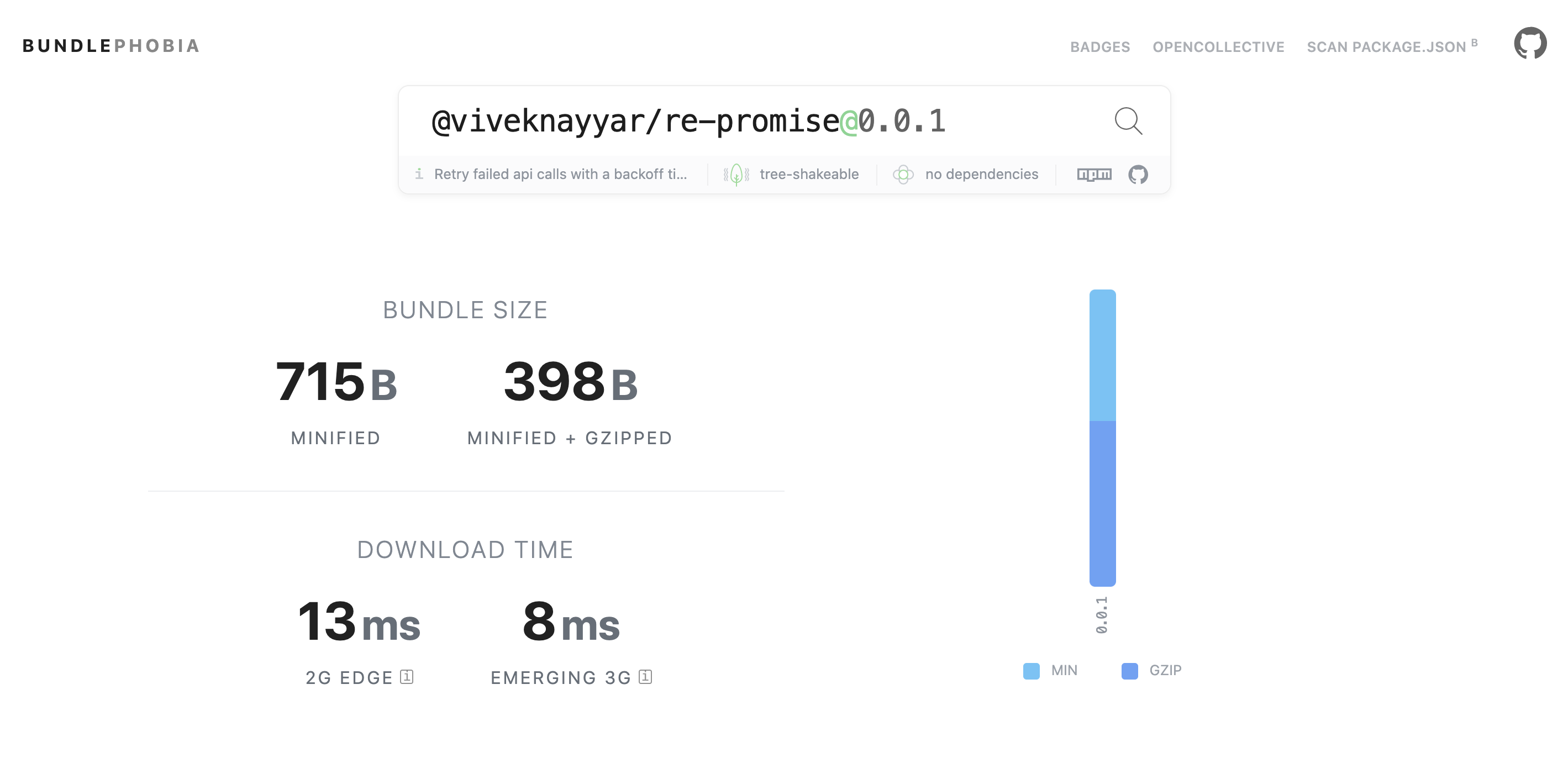
π Contribute
Show your β€οΈ and support by giving a β. Any suggestions and pull request are welcome !
π License
MIT Β© viveknayyar
π· TODO
- Complete README
- Add Examples and Demo
- Test Suite
π Contributors
Thanks goes to these wonderful people (emoji key):
Vivek Nayyar π¬ π π» π¨ π π€ π π§ |
This project follows the all-contributors specification. Contributions of any kind welcome!
Contributors β¨
Thanks goes to these wonderful people (emoji key):
This project follows the all-contributors specification. Contributions of any kind welcome!