README
About
Module will allow you to synchronously or asynchronously import (requires) all modules from the folder you specify.
You can use modules from the returned object, or you can invoke function per file
Installation
npm i directory-import
After install, you can require module and import files:
const importDir = require('index');
// Returns: { filePath1: fileData1, filePath2: fileData2, ... },
const importedModules = importDir({ directoryPath: './' });
Simple usage
This is one simple example of how to use the library and how it works under the hood:
const importDir = require('index');
const importedModules = importDir({ directoryPath: '../sample-directory' });
console.info(importedModules);
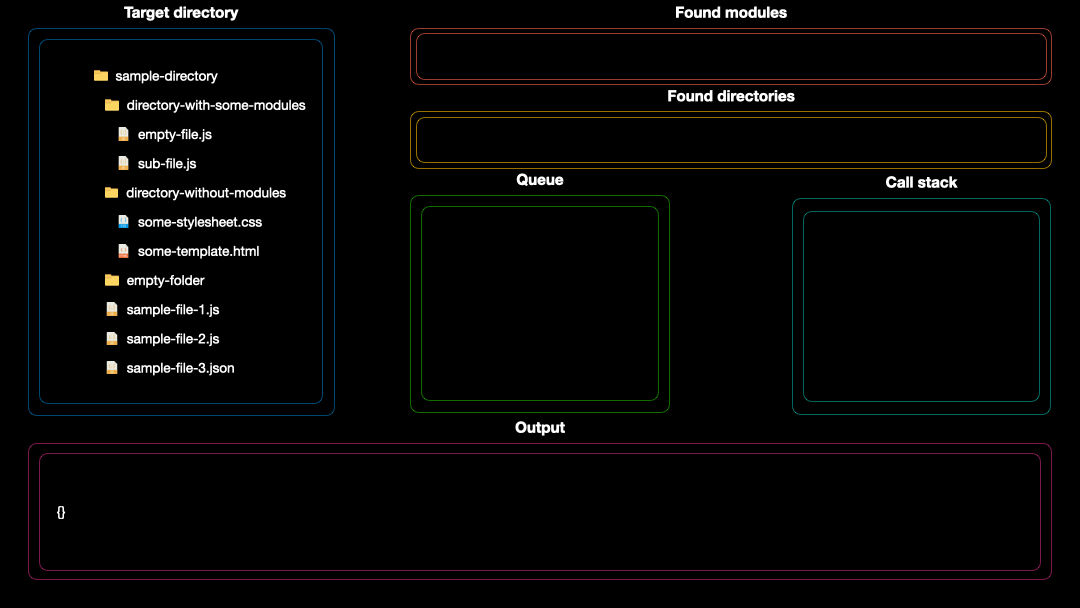
Path to directory from GIF above
You can invoke callback on each file
This can be useful when, for example, you need to do some action depending on the imported file.
const importDir = require('index');
importDir({ directoryPath: '../sample-directory' }, (moduleName, modulePath, moduleData) => {
console.info({ moduleName, modulePath, moduleData });
});
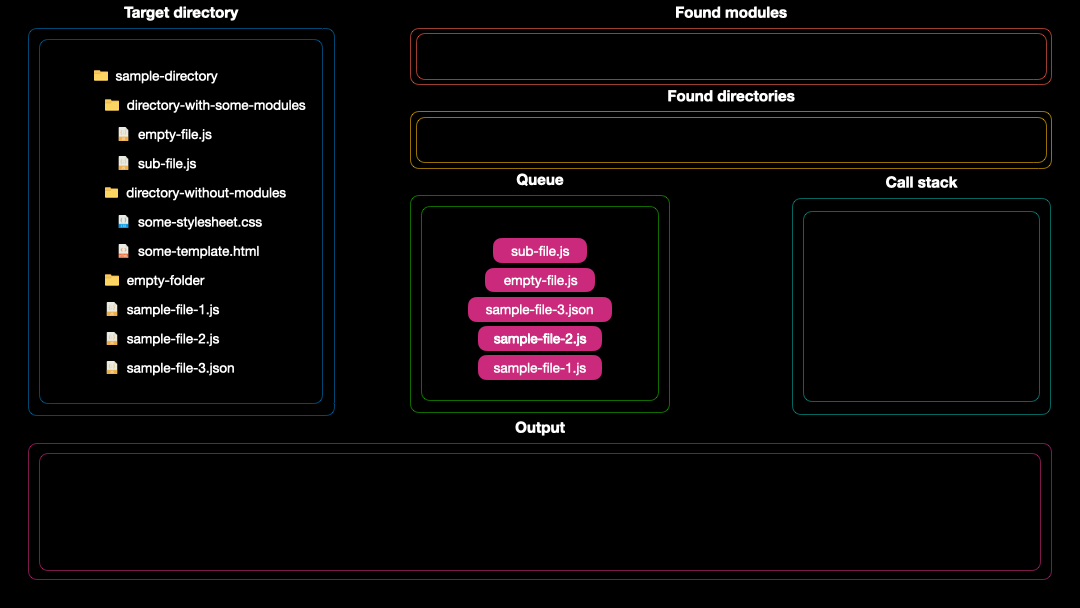
Params
{Object} Options:
Property | Type | Default value | Description |
---|---|---|---|
directoryPath | String | "./" | Relative path to directory |
importMethod | String | "sync" | Import files synchronously, or asynchronously |
includeSubdirectories | Boolean | true | If false — files in subdirectories will not be imported |
webpack | Boolean | false | Webpack support. Example using |
limit | Number | 0 | Indicates how many files to import. 0 - to disable the limit |
exclude | RegExp | undefined | Exclude files paths. Example |
{Function} Callback:
Property | Type | Description |
---|---|---|
fileName | String | File name |
filePath | String | File path |
fileData | String | Exported file data |
More examples
Minimum code to run modules that are in the same folder as the code below:
const importDir = require('directory-import');
importDir();
Async call:
const importDir = require('directory-import');
const importedModules = importDir({ importMethod: 'async', directoryPath: '../sample-directory' });
// Promise { <pending> }
console.info(importedModules);
Async call with callback:
const importDir = require('directory-import');
importDir({ importMethod: 'async', directoryPath: '../sample-directory' }, (moduleName, modulePath, moduleData) => {
// {
// moduleName: 'sample-file-1',
// modulePath: '/sample-file-1.js',
// moduleData: 'This is first sampleFile'
// }
// ...
console.info({ moduleName, modulePath, moduleData });
});
Put the result in a variable and invoke a callback for each module
const importDir = require('directory-import');
const importedModules = importDir({ directoryPath: '../sample-directory' }, (moduleName, modulePath, moduleData) => {
// {
// moduleName: 'sample-file-1',
// modulePath: '/sample-file-1.js',
// moduleData: 'This is first sampleFile'
// }
// ...
console.info({ moduleName, modulePath, moduleData });
});
// {
// '/sample-file-1.js': 'This is first sampleFile',
// ...
// }
console.info(importedModules);
Exclude .json extension
const importDir = require('directory-import');
const result = importDir({ directoryPath: '../sample-directory', exclude: /.json$/g });
console.info(result);
using with webpack
// You must specify the node_modules dir. Otherwise webpak will generate an error
const importDir = require('node_modules/directory-import');
// or const importDir = require('../node_modules/directory-import');
// You must specify the path from the root directory.
// And also indicate that we want to work with the webpack (webpack: true)
const result = importDir({ directoryPath: './sample-directory', webpack: true });
console.info(result);
Help
If you don't understand something in the documentation, you are experiencing problems, or you just need a gentle nudge in the right direction, please don't hesitate to join our official Discord server.
Although the server was created for Russian speakers, you can also write in English! We will understand you!