README
Electron Preferences
Introduction
This package provides Electron developers with a simple, consistent interface for managing user preferences. It includes two primary components:
- A GUI interface within which the users of your application can manage their preferences.
- An API for interacting with the service.
Using the API, developers can:
- Define default preferences
- Read / write values on demand
- Define the layout of the preferences window.
To see the library in action, clone this repository and see the demo application that is included within the example
folder:
$ git clone https://github.com/tkambler/electron-preferences.git
$ cd electron-preferences
$ npm i
$ npm run build
$ npm run example
$ npm run lint
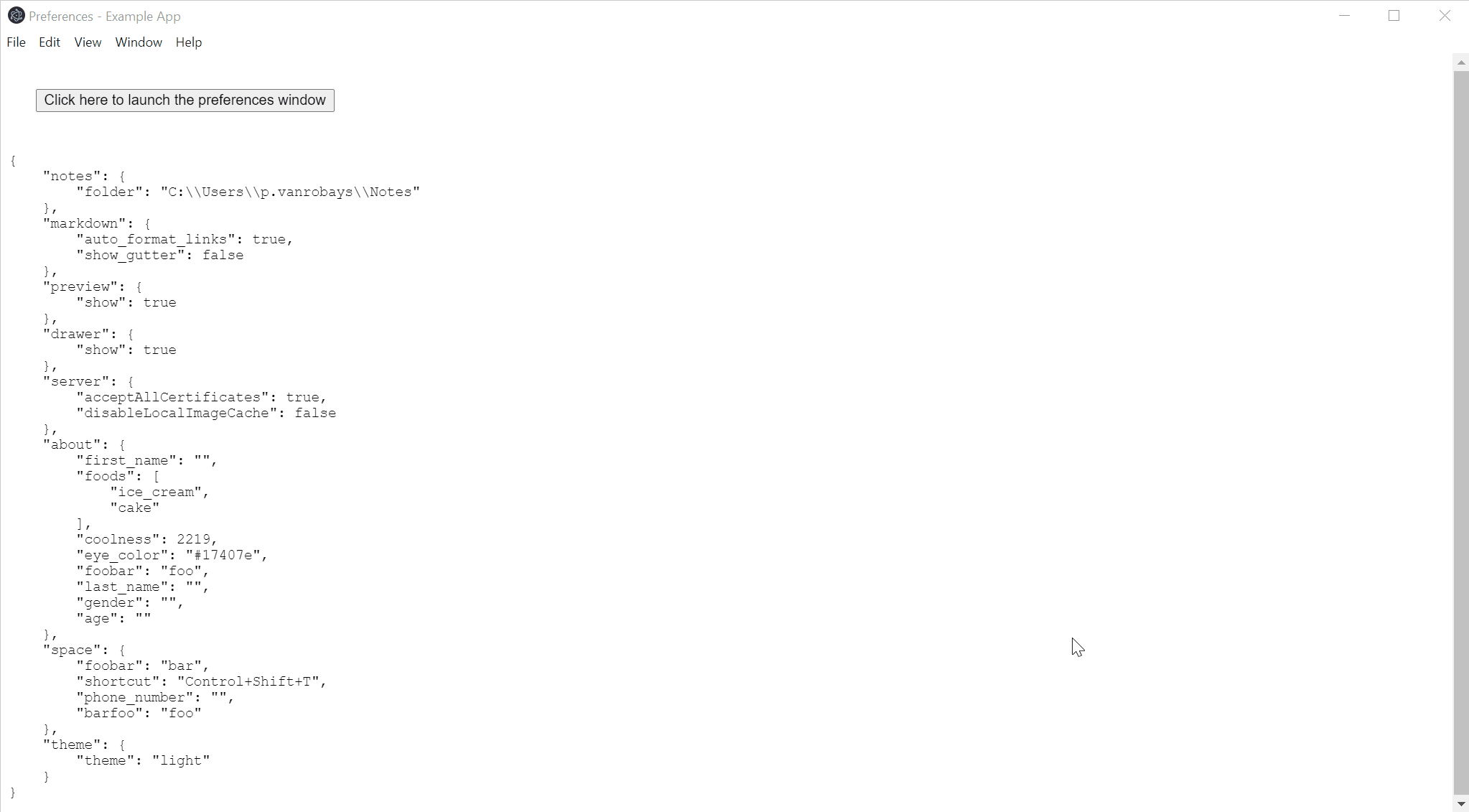
Getting Started
Initializing the Preferences Service
Within your application's main process, create a new instance of the ElectronPreferences
class, as shown below.
Please keep in mind that not all available components are used here. For an example usage of them, check out example/preferences.js
const electron = require('electron');
const app = electron.app;
const path = require('path');
const os = require('os');
const ElectronPreferences = require('electron-preferences');
// import ElectronPreferences from 'electron-preferences' //Or if you prefer to use module imports
const preferences = new ElectronPreferences({
/**
* Where should preferences be saved?
*/
'dataStore': path.resolve(app.getPath('userData'), 'preferences.json'),
/**
* Default values.
*/
'defaults': {
'notes': {
'folder': path.resolve(os.homedir(), 'Notes')
},
'markdown': {
'auto_format_links': true,
'show_gutter': false
},
'preview': {
'show': true
},
'drawer': {
'show': true
}
},
/**
* The preferences window is divided into sections. Each section has a label, an icon, and one or
* more fields associated with it. Each section should also be given a unique ID.
*/
'sections': [
{
'id': 'about',
'label': 'About You',
/**
* See the list of available icons below.
*/
'icon': 'single-01',
'form': {
'groups': [
{
/**
* Group heading is optional.
*/
'label': 'About You',
'fields': [
{
'label': 'First Name',
'key': 'first_name',
'type': 'text',
/**
* Optional text to be displayed beneath the field.
*/
'help': 'What is your first name?'
},
{
'label': 'Last Name',
'key': 'last_name',
'type': 'text',
'help': 'What is your last name?'
},
{
'label': 'Gender',
'key': 'gender',
'type': 'dropdown',
'options': [
{'label': 'Male', 'value': 'male'},
{'label': 'Female', 'value': 'female'},
{'label': 'Unspecified', 'value': 'unspecified'},
],
'help': 'What is your gender?'
},
{
'label': 'Which of the following foods do you like?',
'key': 'foods',
'type': 'checkbox',
'options': [
{ 'label': 'Ice Cream', 'value': 'ice_cream' },
{ 'label': 'Carrots', 'value': 'carrots' },
{ 'label': 'Cake', 'value': 'cake' },
{ 'label': 'Spinach', 'value': 'spinach' }
],
'help': 'Select one or more foods that you like.'
},
{
'label': 'Coolness',
'key': 'coolness',
'type': 'slider',
'min': 0,
'max': 9001
},
{
'label': 'Eye Color',
'key': 'eye_color',
'type': 'color',
'format': 'hex', // can be hex, hsl or rgb
'help': 'Your eye color'
}
]
}
]
}
},
{
'id': 'notes',
'label': 'Notes',
'icon': 'folder-15',
'form': {
'groups': [
{
'label': 'Stuff',
'fields': [
{
'label': 'Read notes from folder',
'key': 'folder',
'type': 'directory',
'help': 'The location where your notes will be stored.',
'multiSelections': false,
'noResolveAliases': false,
'treatPackageAsDirectory': false,
'dontAddToRecent': true
},
{
'label': 'Select some images',
'key': 'images',
'type': 'file',
'help': 'List of selected images',
'filters': [
{ name: 'Joint Photographic Experts Group (JPG)', extensions: ['jpg', 'jpeg', 'jpe', 'jfif', 'jfi', 'jif'] },
{ name: 'Portable Network Graphics (PNG)', extensions: ['png'] },
{ name: 'Graphics Interchange Format (GIF)', extensions: ['gif'] },
{ name: 'All Images', extensions: ['jpg', 'jpeg', 'jpe', 'jfif', 'jfi', 'jif', 'png', 'gif'] },
//{ name: 'All Files', extensions: ['*'] }
],
'multiSelections': true, //Allow multiple paths to be selected
'showHiddenFiles': true, //Show hidden files in dialog
'noResolveAliases': false, //(macos) Disable the automatic alias (symlink) path resolution. Selected aliases will now return the alias path instead of their target path.
'treatPackageAsDirectory': false, //(macos) Treat packages, such as .app folders, as a directory instead of a file.
'dontAddToRecent': true //(windows) Do not add the item being opened to the recent documents list.
},
{
'label': 'Other Settings',
'fields': [
{
'label': "Foo or Bar?",
'key': 'foobar',
'type': 'radio',
'options': [
{'label': 'Foo', 'value': 'foo'},
{'label': 'Bar', 'value': 'bar'},
{'label': 'FooBar', 'value': 'foobar'},
],
'help': 'Foo? Bar?'
}
]
},
{
'heading': 'Important Message',
'content': '<p>The quick brown fox jumps over the long white fence. The quick brown fox jumps over the long white fence. The quick brown fox jumps over the long white fence. The quick brown fox jumps over the long white fence.</p>',
'type': 'message',
}
]
}
]
}
},
{
'id': 'lists',
'label': 'Lists',
'icon': 'notes',
'form': {
'groups': [
{
'label': 'Lists',
'fields': [
{
'label': 'Favorite foods',
'key': 'foods',
'type': 'list',
'size': 15,
'help': 'A list of your favorite foods',
'addItemValidator': /^[A-Za-z ]+$/.toString(),
'addItemLabel': 'Add favorite food'
},
{
'label': 'Best places to visit',
'key': 'places',
'type': 'list',
'size': 10,
'style': {
'width': '75%'
},
'help': 'An ordered list of nice places to visit',
'orderable': true
}
]
}
]
}
},
{
'id': 'space',
'label': 'Other Settings',
'icon': 'spaceship',
'form': {
'groups': [
{
'label': 'Other Settings',
'fields': [
{
'label': "Foo or Bar?",
'key': 'foobar',
'type': 'radio',
'options': [
{'label': 'Foo', 'value': 'foo'},
{'label': 'Bar', 'value': 'bar'},
{'label': 'FooBar', 'value': 'foobar'},
],
'help': 'Foo? Bar?'
}
]
}
]
}
}
],
/**
* These parameters on the preference window settings can be overwrinten
*/
browserWindowOpts: {
'title': 'My custom preferences title',
'width': 900,
'maxWidth': 1000,
'height': 700,
'maxHeight': 1000,
'resizable': true,
'maximizable': false,
//...
},
/**
* These parameters create an optional menu bar
*/
menu: Menu.buildFromTemplate(
[
{
label: 'Window',
role: 'window',
submenu: [
{
label: 'Close',
accelerator: 'CmdOrCtrl+W',
role: 'close'
}
]
}
]
),
/**
* If you want to apply your own CSS. The path should be relative to your appPath.
*/
css: 'custom-style.css'
});
Interacting with the Preferences Service from the Main Process
// Show the preferences window on demand.
preferences.show();
// Get a value from the preferences data store
const myPref = preferences.value('some.nested.key');
// Save a value within the preferences data store
preferences.value('some.nested.key', 'my-value');
// Subscribing to preference changes.
preferences.on('save', (preferences) => {
console.log(`Preferences were saved.`, JSON.stringify(preferences, null, 4));
});
Interacting with the Preferences Service from the Renderer Process
const { ipcRenderer, remote } = require('electron');
// Fetch the preferences object
const preferences = ipcRenderer.sendSync('getPreferences');
// Display the preferences window
ipcRenderer.send('showPreferences');
// Listen to the `preferencesUpdated` event to be notified when preferences are changed.
ipcRenderer.on('preferencesUpdated', (e, preferences) => {
console.log('Preferences were updated', preferences);
});
// Instruct the preferences service to update the preferences object from within the renderer.
ipcRenderer.sendSync('setPreferences', { ... });
Dark or Light? 🌓
You prefer a dark theme over a light theme? No problem, we have them both. The library will use whatever theme you're using with Electron. See the example on how to add the option to your preferences.
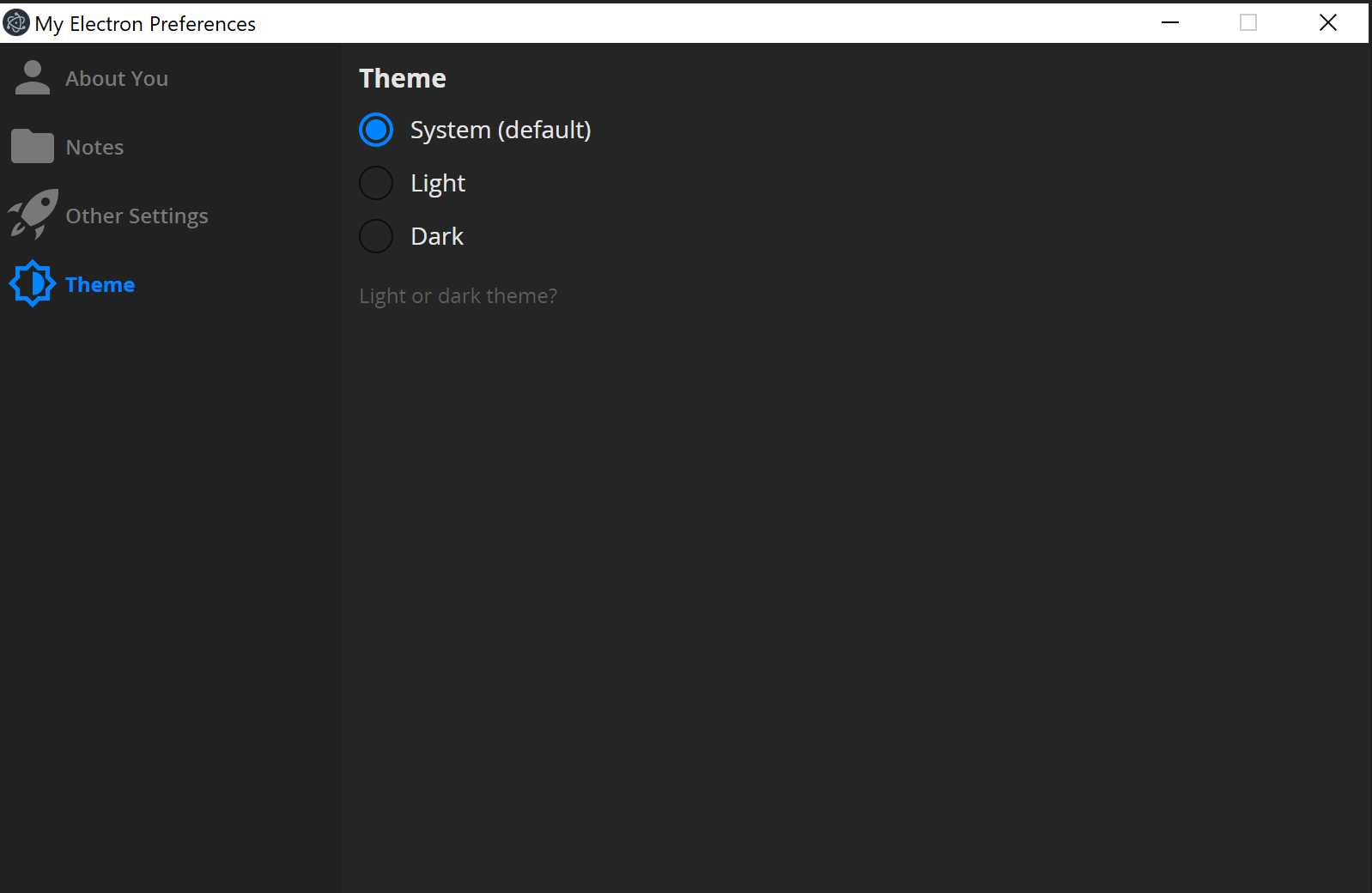
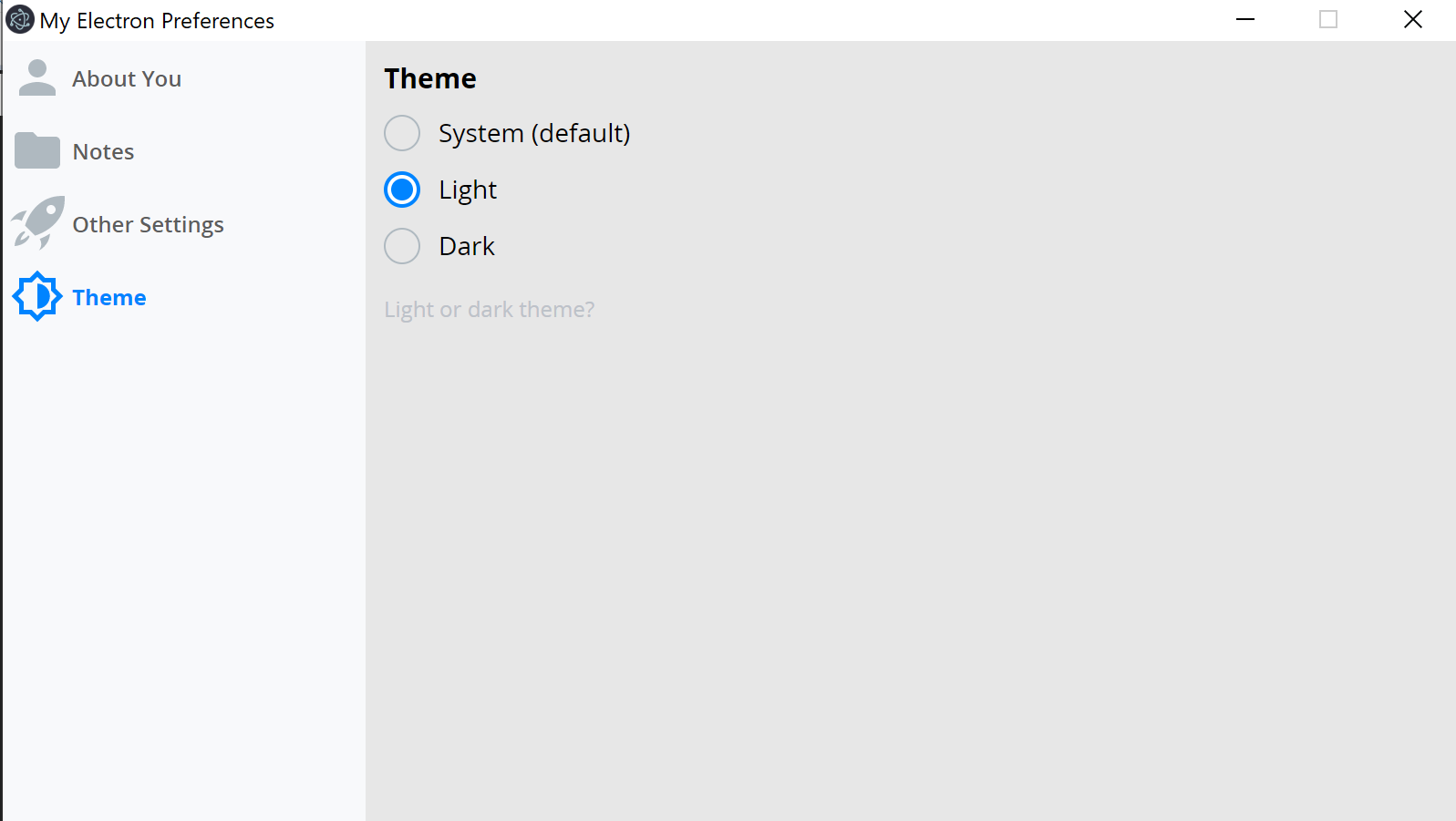
Still not matching your layout? You can easily customize the complete look by injecting your own custom CSS!
Field Types
The library includes built-in support for the following field types:
- Text
- Dropdown
- Message
- Folder/File selection
- Checkbox
- Radio
- Slider
- (Ordered) Lists
- Accelerator (for shortcut input)
- Color picker
Adding support for additional field types if easy, if you're familiar with React. PR's for such additions are welcome.
Icons
The following icons come packaged with the library and can be specified when you define the layout of your preferences window.
Name | Icon |
---|---|
archive-2 | |
archive-paper | |
award-48 | |
badge-13 | |
bag-09 | |
barcode-qr | |
bear-2 | |
bell-53 | |
bookmark-2 | |
brightness-6 | |
briefcase-24 | |
calendar-60 | |
camera-20 | |
cart-simple | |
chat-46 | |
check-circle-07 | |
cloud-26 | |
compass-05 | |
dashboard-level | |
diamond | |
edit-78 | |
email-84 | |
eye-19 | |
favourite-31 | |
flag-points-32 | |
flash-21 | |
folder-15 | |
gift-2 | |
grid-45 | |
handout | |
heart-2 | |
home-52 | |
image | |
key-25 | |
layers-3 | |
like-2 | |
link-72 | |
lock-open | |
lock | |
multiple-11 | |
notes | |
pencil | |
phone-2 | |
preferences | |
send-2 | |
settings-gear-63 | |
single-01 | |
single-folded-content | |
skull-2 | |
spaceship | |
square-download | |
square-upload | |
support-16 | |
trash-simple | |
turtle | |
vector | |
video-66 | |
wallet-43 | |
widget | |
world | |
zoom-2 |