README
epicfail
Better error reporting for Node.js command-line apps.
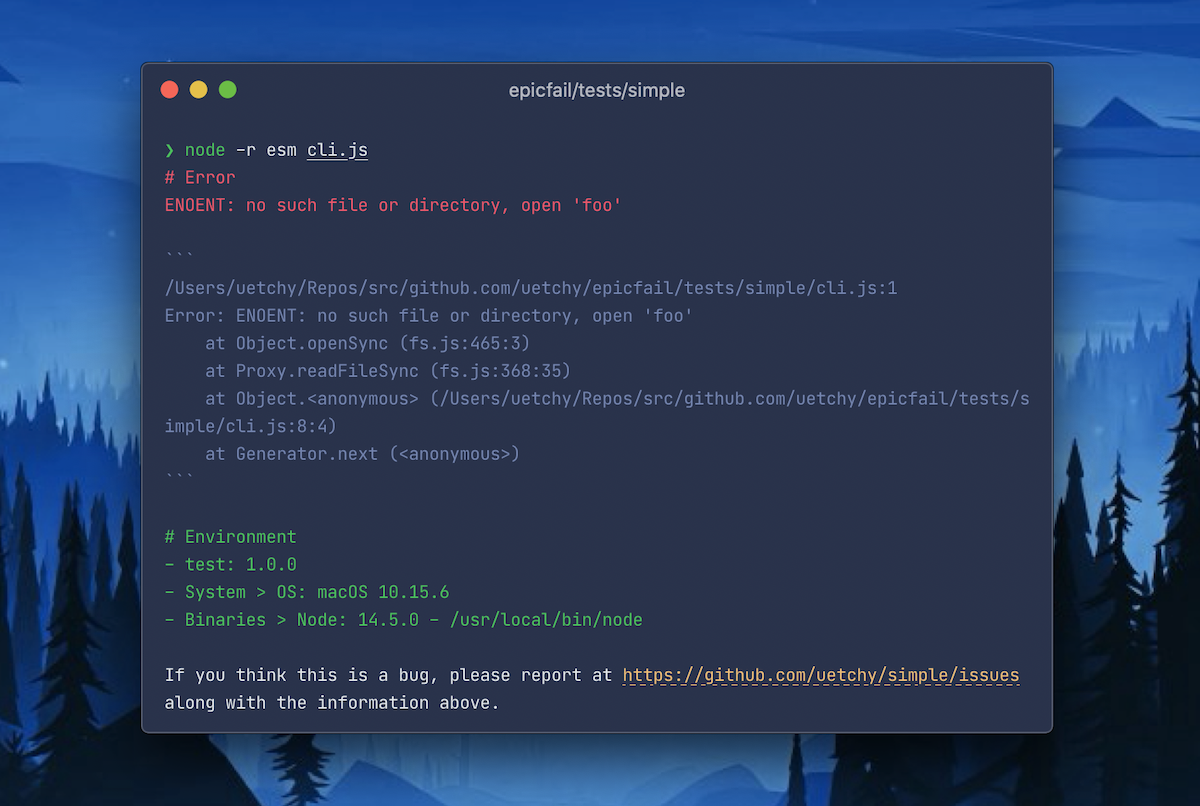
Features
epicfail converts
unhandledRejection
anduncaughtException
into graceful and helpful error message for both users and developers.
⬇️ Prints error messages in copy and paste ready Markdown.
🌐 Asks users to report a bug (navigate users to bugs.url
in package.json
).
🍁 Shows machine environments (OS, Node.js version, etc).
👀 Suggests related issues in GitHub.
🛠 Integration with error aggregation service (like Sentry).
Table of Contents
Install
npm install --save epicfail
# or
yarn add epicfail
Use
ESModules
import { epicfail } from "epicfail";
epicfail(import.meta.url);
// your CLI app code goes here
fs.readFileSync("foo"); // => will cause "ENOENT: no such file or directory, open 'foo'"
CommonJS
const { epicfail } = require("epicfail");
epicfail(require.main.filename);
// your CLI app code goes here
fs.readFileSync("foo"); // => will cause "ENOENT: no such file or directory, open 'foo'"
Options
true
)
stacktrace (default: Show stack trace.
import { epicfail } from "epicfail";
epicfail(import.meta.url, {
stacktrace: false,
});
false
)
issues (default: Search and show related issues in GitHub Issues.
import { epicfail } from "epicfail";
epicfail(import.meta.url, {
issues: true,
});
env
Show environment information. You can find all possible options here. Set to false
to disable it.
import { epicfail } from "epicfail";
epicfail(import.meta.url, {
env: {
System: ["OS", "CPU"],
Binaries: ["Node", "Yarn", "npm"],
Utilities: ["Git"],
},
});
Default values:
{
"System": ["OS"],
"Binaries": ["Node"]
}
true
)
message (default: Show bug tracker URL and ask users to report the error.
import { epicfail } from "epicfail";
epicfail(import.meta.url, { message: false });
() => false
)
assertExpected (default: While processing an error, if assertExpected(error)
returns true
, epicfail just prints the error message without any extra information; which is the same behaviour as the logAndExit()
function described below.
import { epicfail } from "epicfail";
epicfail(import.meta.url, {
assertExpected: (err) => err.name === "ArgumentError",
});
undefined
)
onError (default: Pass the function that process the error and returns event id issued by external error aggregation service.
import { epicfail } from "epicfail";
import Sentry from "@sentry/node";
epicfail(import.meta.url, {
onError: (err) => Sentry.captureException(err), // will returns an event id issued by Sentry
});
Advanced Usage
Print error message without extra information
Use logAndExit()
to print error message in red text without any extra information (stack trace, environments, etc), then quit program. It is useful when you just want to show the expected error message without messing STDOUT around with verbose log messages.
import { epicfail, logAndExit } from "epicfail";
epicfail(import.meta.url);
function cli(args) {
if (args.length === 0) {
logAndExit("usage: myapp <input>");
}
}
cli(process.argv.slice(2));
You can also pass an Error instance:
function cli(args) {
try {
someFunction();
} catch (err) {
logAndExit(err);
}
}
Sentry integration
import { epicfail } from "epicfail";
import Sentry from "@sentry/node";
epicfail(import.meta.url, {
stacktrace: false,
env: false,
onError: Sentry.captureException, // will returns event_id issued by Sentry
});
Sentry.init({
dsn: "<your sentry token here>",
defaultIntegrations: false, // required
});
// your CLI app code goes here
fs.readFileSync("foo"); // => will cause "ENOENT: no such file or directory, open 'foo'"
Runtime options
import {epicfail} from 'epicfail';
epicfail(import.meta.url);
// 1. Use epicfail property in Error instance.
const expected = new Error('Wooops');
expected.epicfail = { stacktrace: false, env: false, message: false };
throw expected;
// 2. Use fail method
import { fail } from 'epicfail';
fail('Wooops', { stacktrace: false, env: false, message: false });
// 3. Use EpicfailError class (useful in TypeScript)
import { EpicfailError } from 'epicfail';
const err = new EpicfailError('Wooops', { stacktrace: false, env: false, message: false };);
throw err;