README
github-now-playing
📖 Table of Contents
Motivation
GitHub introduced a new feature that allows you to set a status on your profile, so I thought it would be a cool idea if I could share the music I'm listening to — kind of like #NowPlaying — right on my GitHub profile!
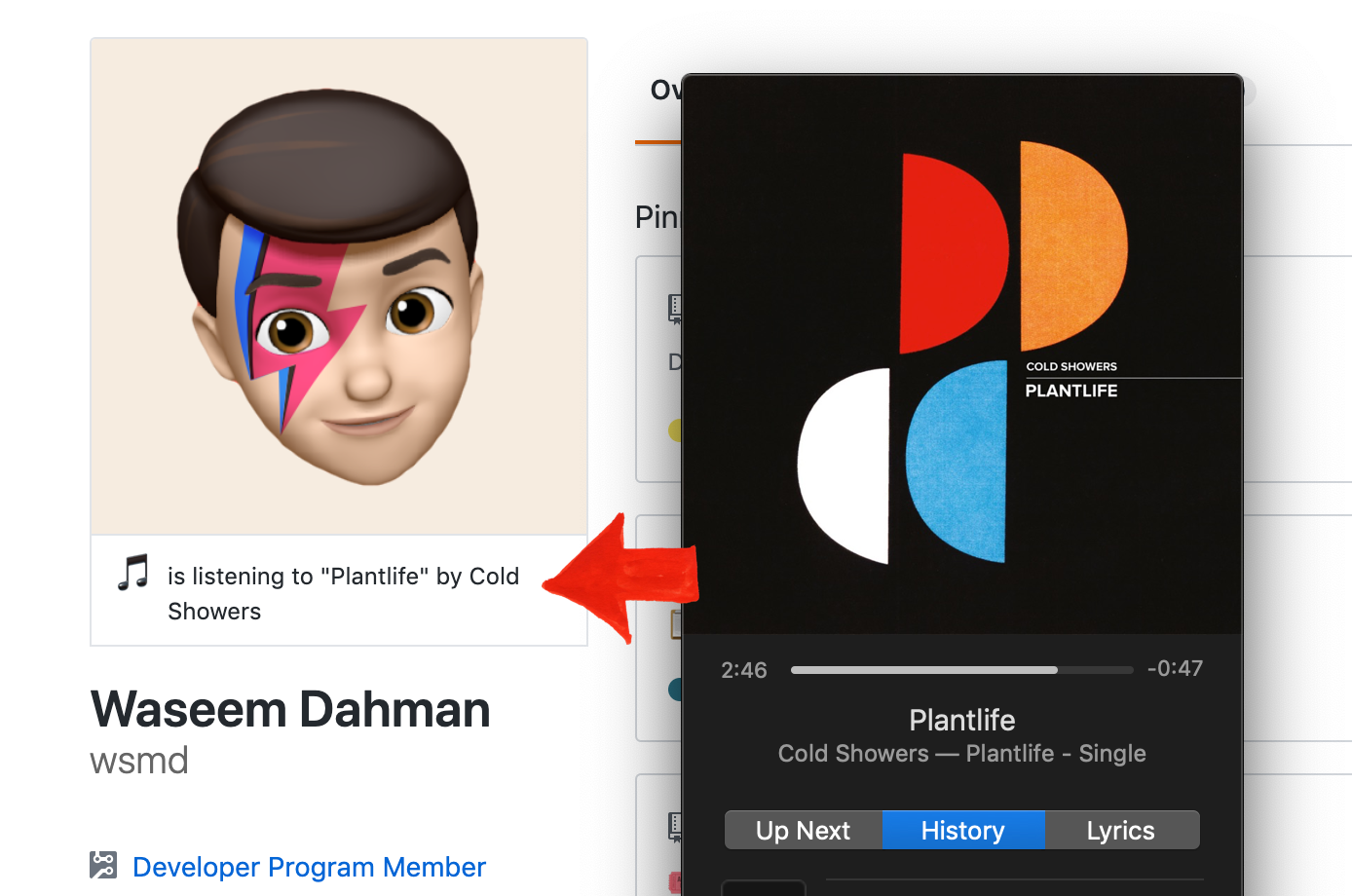
Installation
This library is available on the npm registry as a node module and can be installed by running:
# via npm
npm install --save github-now-playing
# via yarn
yarn add github-now-playing
You also need to generate a personal access token with the user scope to allow this library to communicate with GitHub.
Example
import { GitHubNowPlaying } from 'github-now-playing';
const nowPlaying = new GitHubNowPlaying({
token: process.env.GITHUB_ACCESS_TOKEN,
});
// Create a new source to retrieve the track that is currently playing
const spotifySource = new GitHubNowPlaying.Sources.Spotify({
// wait time in milliseconds between checks for any track changes
updateFrequency: 1000,
});
// Make sure a source is set before calling listen()
nowPlaying.setSource(spotifySource);
// Don't forget to handle the error event!
nowPlaying.on(GitHubNowPlaying.Events.Error, error => {
console.log('something went wrong');
});
// Listen to any track changes and update the profile status accordingly.
nowPlaying.listen();
// Don't forget to stop reporting any track changes when the process exists.
process.on('SIGINT', () => {
// Calling the stop() method will clear the profile status.
nowPlaying.stop()
});
API
github-now-playing
exposes a named export class GitHubNowPlaying
that is also a namespace for various source providers and event names.
GitHubNowPlaying
Class: new GitHubNowPlaying(options: GitHubNowPlayingConstructorOptions)
Creates a new instance of GitHubNowPlaying
.
Constructor Options
An object with the following keys:
token: string
: a personal access token with the user scope.
Methods
nowPlaying.setSource(source)
Assigns a source provider object from which the currently-playing track will be retrieved.
This method must be called before calling listen()
.
nowPlaying.on(event, listener)
Adds the listener
function as an event handler for the named event
. The event
parameter can be one of the values available under the GitHubNowPlaying.Events
namespace.
Returns a reference to the GitHubNowPlaying
instance, so that calls can be chained.
nowPlaying.off(event, listener)
Removes the specified listener
function for the named event
.
The event
parameter can be one of the values available under the GitHubNowPlaying.Events
namespace.
Returns a reference to the GitHubNowPlaying
instance, so that calls can be chained.
nowPlaying.listen()
Starts listening to any track changes coming from the specified source and updates the GitHub profile status accordingly.
The event GitHubNowPlaying.Events.ListenStart
is emitted upon calling this method.
Additionally, every time the profile status is updated, the event GitHubNowPlaying.Events.StatusUpdated
is emitted with the profile status object.
Note that upon calling listen()
, the profile status will be updated immediately to reflect the currently playing track.
nowPlaying.stop()
Stops listening to any track changes.
Calling this method will result in clearing the profile status if it has been already updated with a currently playing track.
If the status is cleared, the event GitHubNowPlaying.Events.StatusCleared
is emitted, then followed by the event GitHubNowPlaying.Events.ListenStop
.
This method is asynchronous and will resolve after clearing the profile status.
GitHubNowPlaying.Sources
Sources Providers: GitHubNowPlaying
relies on a source provider object that retrieves the currently playing track from a specific source.
These sources can be either local desktop applications, such iTunes or Spotify, or even via web APIs, such as Last.fm.
GitHubNowPlaying
comes with built-in support for all of these sources. Note that support for desktop applications is currently limited to macOS.
The following sources are available under the namespace GitHubNowPlaying.Sources
:
LastFM
Fetches information about the currently-playing track via Last.fm.
const lastFmSource = new GitHubNowPlaying.Sources.LastFM({
apiKey: process.env.LAST_FM_API_KEY, // Your Last.fm API key
updateFrequency: 1000,
});
nowPlaying.setSource(lastFmSource);
ITunes
Fetches information about the currently-playing track in iTunes.
const iTunesSource = new GitHubNowPlaying.Sources.ITunes({
updateFrequency: 1000,
});
nowPlaying.setSource(iTunesSource);
Platforms supported: macOS
Spotify
Fetches information about the currently-playing track in Spotify.
const spotifySource = new GitHubNowPlaying.Sources.Spotify({
updateFrequency: 1000,
});
nowPlaying.setSource(spotifySource);
Platforms supported: macOS
GitHubNowPlaying.Events
Events: Instances of GitHubNowPlaying
emit various events during the program life-cycle. You can add or remove event listeners via on()
and off()
respectively.
Error
Emitted when an error occurs:
nowPlaying.on(GitHubNowPlaying.Events.Error, (error) => { /* ... */ });
ListenStart
Emitted when GitHubNowPlaying
starts listening to track changes via the specified source:
nowPlaying.on(GitHubNowPlaying.Events.ListenStart, () => { /* ... */ });
ListenStop
Emitted when GitHubNowPlaying
has stopped listening to track changes via the specified source:
nowPlaying.on(GitHubNowPlaying.Events.ListenStop, () => { /* ... */ });
StatusUpdated
Emitted when GitHubNowPlaying
has updated the profile status with currently-playing track successfully. Listeners of this event are called with the user-status object.
nowPlaying.on(GitHubNowPlaying.Events.ListenStop, (status) => { /* ... */ });
StatusCleared
Emitted when GitHubNowPlaying
has cleared the profile status after it has been updated with a currently playing track. This happens when the source provider reports that there are no tracks are currently playing, or when stop()
is called.
nowPlaying.on(GitHubNowPlaying.Events.StatusCleared, () => { /* ... */ });
See Also
License
MIT