README
GS - Car Fleet Management (CFM)
Tested environment config
- Node 10.6.0
- NPM 6.1.0
Run the app
- Rename
env.sample
file to.env
npm install
npm run dev
(should auto-open a tab)- Otherwise,
localhost:4000
Run the tests
npm run test
Warning: "extensive" thought process documented below.
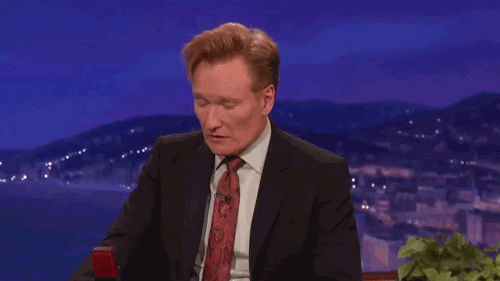
First thoughts
Given the nature of the challenge, my first goal is to spin up an environment that allows me to begin coding in the preferred frameworks.
Tools and utils
When starting a new project, after looking at the requirements, getting a handle on the scope and establishing a framework, my next thought is: "which tools and utilities do I want include in the project to ensure my success."
- Given it's a fairly small project, I will forego Redux and utilize React as much as possible (hooks, etc.)
- Reselect: to provide an easy way to return data from the store
- Immutable: lots of helpers and ensuring ... immutability
- Jest: to handle unit testing and snapshots
- StyledComponents: because I love them, overall
- Let's talk CSS Modules pros/cons later
Setup cont.
- Install tools/utilities and wire up, configs, etc.
- Think through file organization based on requirements
- Where do I want shared/helpers to live?
- How big is the app going to get?
- What things have I done in the past that I don't want to ever experience again?
General process/thinking
- Review requirements and establish a "mock state tree" that meets said requirements
- Enable ECMAScript 6 Imports in Node.JS to handle local store for API
- ESM to the rescue!
(Rough) Technical Specification
Note: numeric values are based on agile's fibonacci scale, to determine estimated time needed to complete a given task
(3) Define and create a RESTful interface
- See requirements document
- Node/Express/Mongoose/MongoDB
(2) Test and seed the data
- Write a seed method to populate the vehicle data (or just use MongoDB directly)
- Write method to insert a "random" set of arbitrary objects into each vehicle's "claimHistory" property
(3) Add an aggregate GET method to REST
- Use Mongoose, to return an aggregate of the following vehicle properties:
- Total count
- Count by year
- Total purchase price
- Use Mongoose, to return an aggregate of the following vehicle properties:
(2) Write a JavaScript version of the aggregate method
- See requirements document
- Do virtually the same thing as the Mongoose aggregate method above
- Return aggregate data for the properties listed above in the client when requested
(2) Wire up Redux and fetch the newly seeded data