README
Middy RDS manager
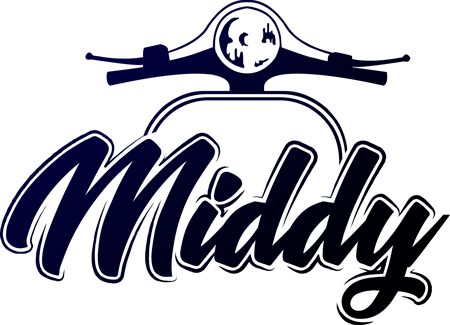
Simple database manager for the middy framework
RDS provides seamless connection with database of your choice.
After initialization your database connection is accessible under:
middy((event, context) => {
const { db } = context;
});
Mind that if you use knex you will also need driver of your choice (check docs), for PostgreSQL that would be:
yarn add pg
// or
npm install pg
Install
To install this middleware you can use NPM:
npm install --save middy-rds
Requires: @middy/core:>=2.0.0
Options
client
(function) (required): client that you want to use when connecting to database of your choice. Designed to be used by knex.js. However, as long as your client is run as client(config), you can use other tools.config
(object) (required): configuration object passed as is to client (knex.js recommended), for more details check knex documentationinternalData
(object) (optional): Pull values from middy internal storage intoconfig.connection
object.cacheKey
(string) (defaultrds
): Cache key for the fetched data responses. Must be unique across all middleware.cachePasswordKey
(string) (defaultrds
):Cache key for the fetched data response related to the password. Must match thecacheKey
for the middleware that stores it.cacheExpiry
(number) (default-1
): How long fetch data responses should be cached for.-1
: cache forever,0
: never cache,n
: cache for n ms.
Note:
config.connection
defaults to:
{
ssl: {
rejectUnauthorized: true,
ca, // rds-ca-2019-root.pem
checkServerIdentity: (host, cert) => {
const error = tls.checkServerIdentity(host, cert)
if (error && !cert.subject.CN.endsWith('.rds.amazonaws.com')) {
return error
}
}
}
}
If your lambda is timing out, likely your database connections are keeping the event loop open. Check out do-not-wait-for-empty-event-loop middleware to resolve this.
Sample usage
Minimal configuration
knex
const rds = require('middy-rds')
const knex = require('knex')
const pg = capturePostgres(require('pg')) // AWS X-Ray
const handler = middy(async (event, context) => {
const { db } = context;
const records = await db.select('*').from('my_table');
console.log(records);
})
.use(rdsSigner({
fetchData: {
rdsToken: {
region: 'ca-central-1',
hostname: '*.ca-central-1.rds.amazonaws.com',
username: 'iam_role',
database: 'postgres',
port: 5432
}
},
cacheKey: 'rds-signer'
}))
.use(rds({
internalData: {
password: 'rdsToken'
},
cacheKey: 'rds',
cachePasswordKey: 'rds-signer',
client: knex,
config: {
client: 'pg',
connection: {
host: '*.ca-central-1.rds.amazonaws.com',
user: 'iam_role',
database: 'postgres',
port: 5432
}
}
}))
pg
const rds = require('middy-rds/pg')
const pg = capturePostgres(require('pg')) // AWS X-Ray
const handler = middy(async (event, context) => {
const { db } = context;
const records = await db.select('*').from('my_table');
console.log(records);
})
.use(rdsSigner({
fetchData: {
rdsToken: {
region: 'ca-central-1',
hostname: '*.ca-central-1.rds.amazonaws.com',
username: 'iam_role',
database: 'postgres',
port: 5432
}
},
cacheKey: 'rds-signer'
}))
.use(rds({
internalData: {
password: 'rdsToken'
},
cacheKey: 'rds',
cachePasswordKey: 'rds-signer',
client: pg.Pool,
config: {
host: '*.ca-central-1.rds.amazonaws.com',
user: 'iam_role',
database: 'postgres'
}
}))
Middy documentation and examples
For more documentation and examples, refers to the main Middy monorepo on GitHub or Middy official website.
Contributing
Everyone is very welcome to contribute to this repository. Feel free to raise issues or to submit Pull Requests.
License
Licensed under MIT License. Copyright (c) 2017-2021 will Farrell and the Middy team.