README
!React: THIS IS NOT REACT
It's a State-Manager (MVC) for Javascript
require('assert')(!React !== React, 'Not-React is Not React');
Methods: createClass, createElement
const notReact = require('not-react');
const nrElement = notReact.createElement(tag, attributes, children);
nrElement.render(); // returns the element html string
const nrClass = notReact.createClass({ render: () => { ... }});
nrClass.setState({ ... }); // returns the class value based on the state
Motivation
I am a fan of simple things. I also like to break things down to make them more understandable. Additionally, I am not a huge fan of being told what not to do. SO. What I have decided to do is make a module that mimics what I think is good in React, but without many of the opinions and yelling. For example: (1) I do not care if you choose to modify the state directly. You probably shouldn't, but who am I to judge your use case. (2) I encourage you to modify the props directly. Yes you can get yourself into trouble, but you are a capable developer right??? It's just Javascript after all.
The Way I See It
The state should only contain the items that when changed will lead to a unique class value. These items should also not be dependent on anything else within the class. If you find yourself in the situation where you have a state change that depends on another state change then you probably need to move that to the props and do your calculating within the stateWillChange lifecycle method. The props are a great place to calculate anything that depends on a state change and ultimately is used to render the class value
Usgae (ES5/ES6 Compatible):
// ///// ///// // ///// ///// // ///// ///// // ///// ///// // ///// /////
// ES6
const hello = require('not-react').createClass({
getInitialState: () => ({ name: 'Louis' }),
render: (state) => `<h1>${state.name}</h1>`
});
hello.setState(); // returns '<h1>Louis</h1>'
hello.setState({name: 'John'}); // returns '<h1>John</h1>'
// ///// ///// // ///// ///// // ///// ///// // ///// ///// // ///// /////
// ES5
var hello = require('not-react').createClass({
getInitialState: function () { return { name: 'Louis' }; },
render: function (state) { return '<h1>' + state.name + '</h1>'; }
});
hello.setState(); // returns '<h1>Louis</h1>'
hello.setState({name: 'John'}); // returns '<h1>John</h1>'
// ///// ///// // ///// ///// // ///// ///// // ///// ///// // ///// /////
REMEMBER!!! Arrow functions droped this binding :( SO: make sure to use traditional functions if you intend to access class properties (like this.props, or this.userDefinedProp)
Gif State Manager Example:
const status = require('not-react').createClass({
getInitialState: () => ({ status: 'pending' }),
getDefaultProps: () => ({
gif: null,
gifs: {
pending: 'http://i.giphy.com/3oEjI6SIIHBdRxXI40.gif',
success: 'http://i.giphy.com/a0h7sAqON67nO.gif',
fail: 'http://i.giphy.com/l0MYEZkOB8xCYM7O8.gif'
}
}),
stateWillChange: function (currentState, nextState) {
this.props.gif = this.props.gifs[nextState.status];
},
render: function () {
// return a markdown gif string
return ''.replace('@gif@', this.props.gif);
}
});
status.setState(); // returns '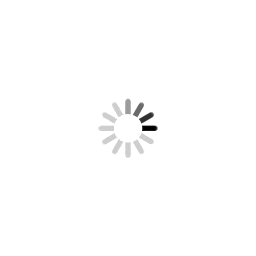'
status.setState({ status: 'fail' }); // returns '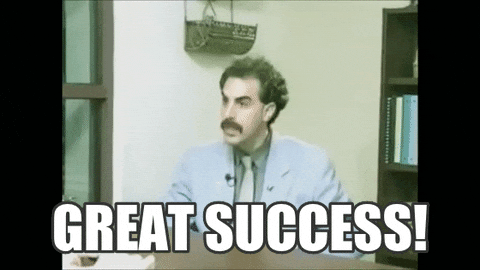'
status.setState({ status: 'success' }); // returns '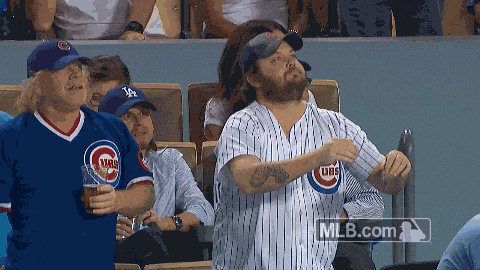'
Initial Values:
- function getInitialState // This method should return the initial class state
- function getDefaultProps // This method should return the initial class props
Lifecycle Methods:
- function setState(state) // The user will invoke the setSate method on the class
- function stateWillChange(currentState, nextState) // The stateWillChange method is called on the class before the render method is called. This is a great place to render the props intended to be used in the render method.
- function render(state) // The main render method will be called and should return the class value based on the state
- function stateDidChange(currentState, previousState) // The stateDidChange method will be called after the render method call stack completes. This is a great place to process logic on the rendered application