README
react-native-rectangle-color-picker
A color picker on diamond or rectangle palette.
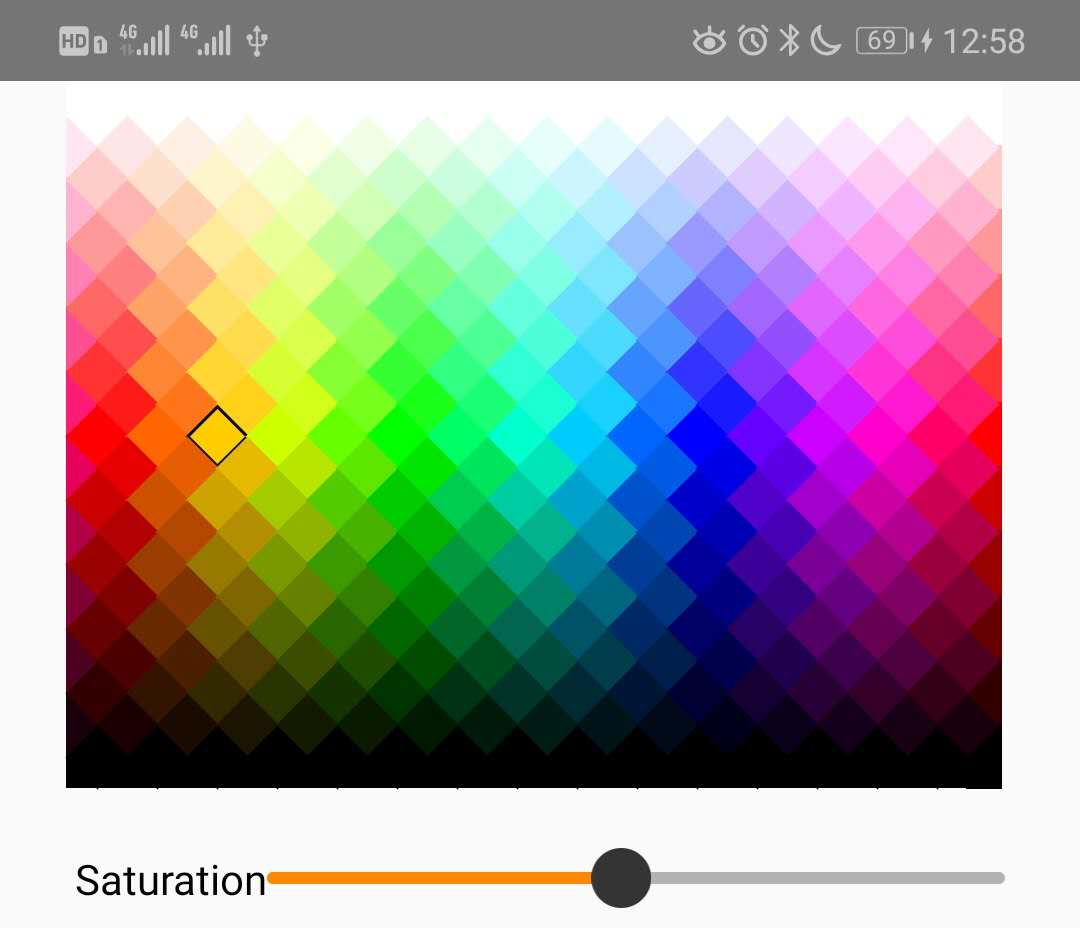
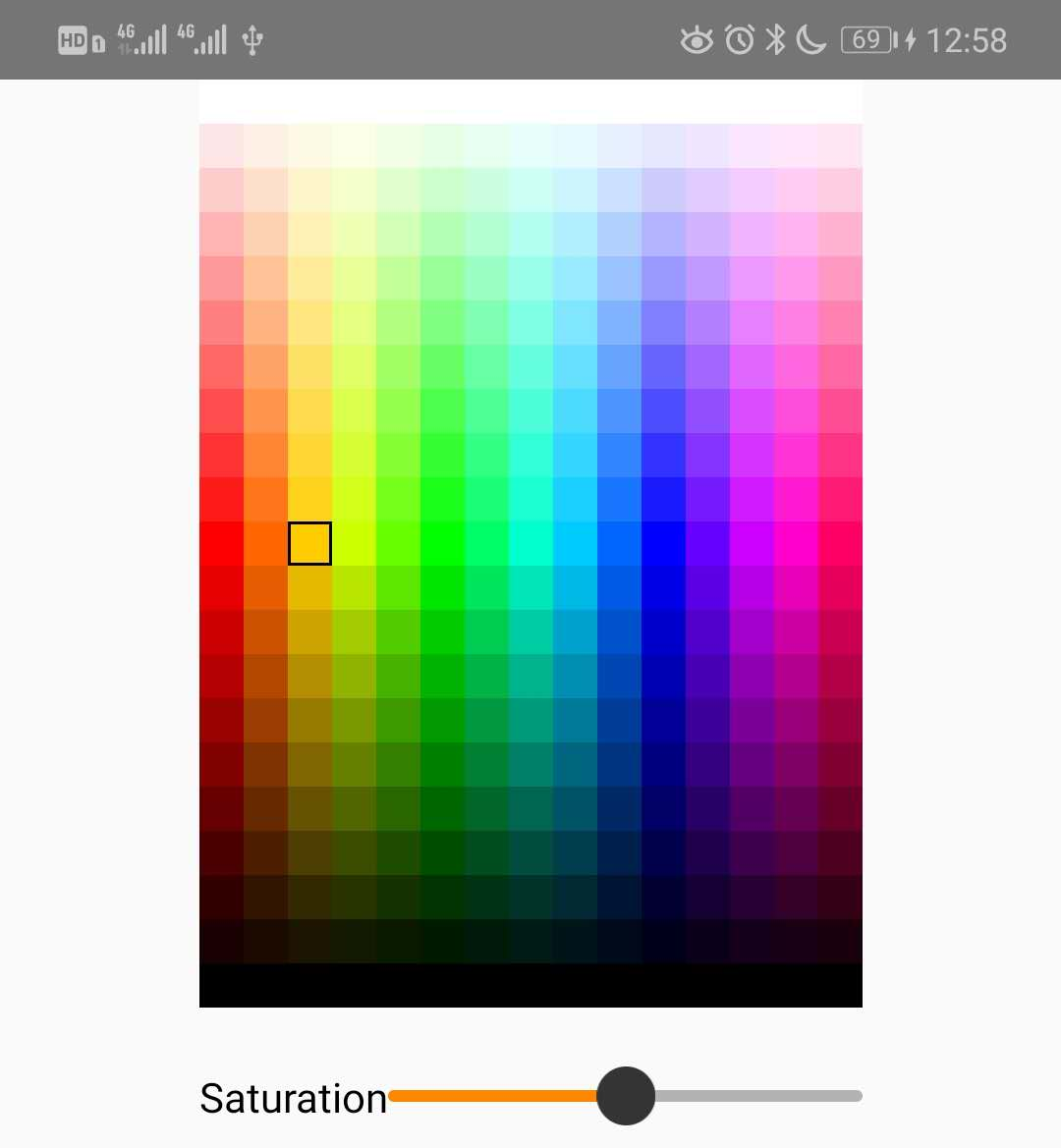
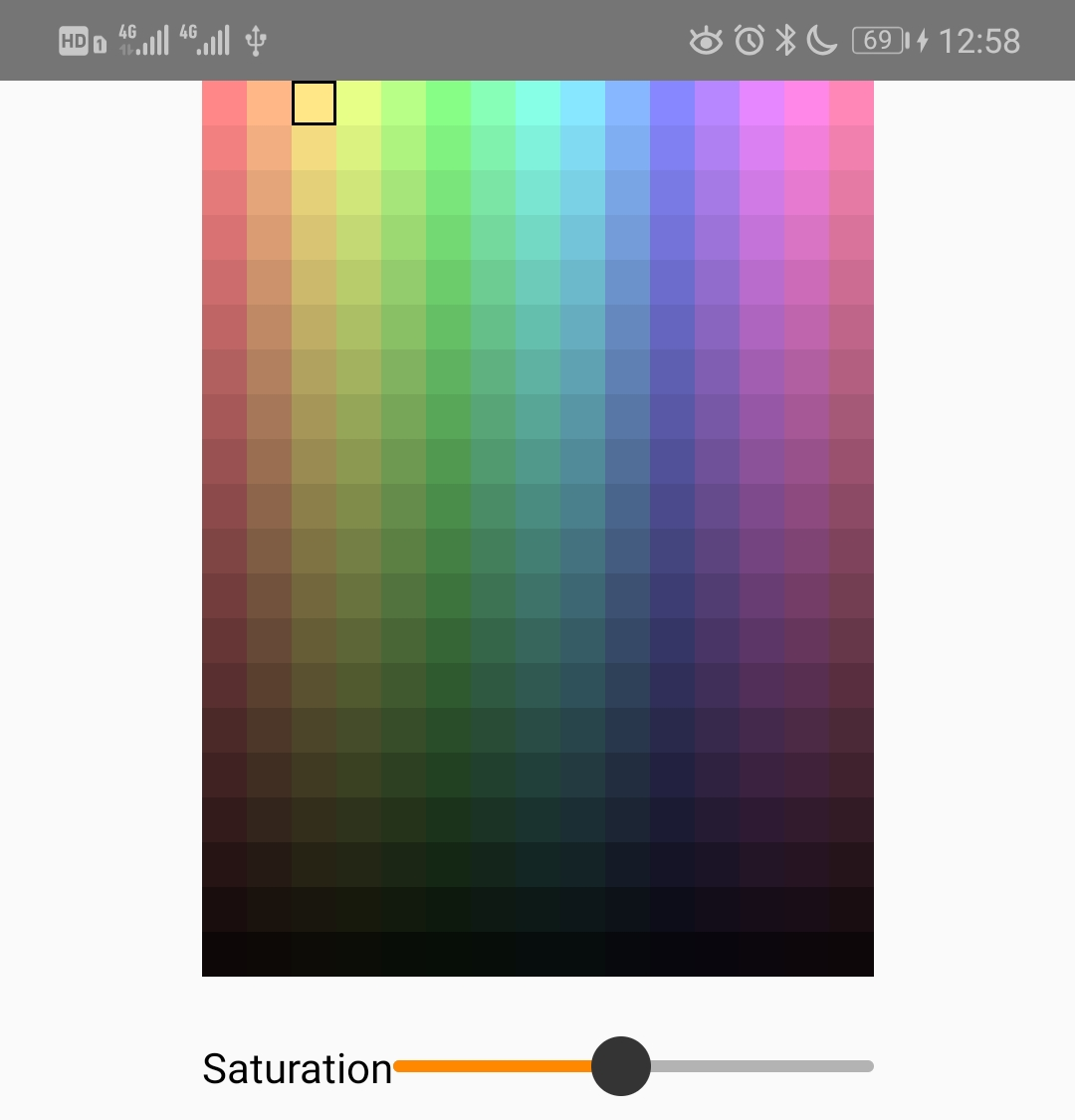
Install
npm i --save react-native-rectangle-color-picker
Usage
import React from 'react';
import { View } from 'react-native';
import tinycolor from 'tinycolor2';
import ColorPicker from 'react-native-rectangle-color-picker';
export default class SliderColorPickerExample extends React.Component {
constructor(props) {
super(props);
this.state = { oldColor: '#dc402b' };
}
componentDidMount() {
setTimeout(() => this.setState({ oldColor: '#fde200' }), 1000);
}
changeColor = colorHsv => this.setState({ oldColor: tinycolor(colorHsv).toHexString() })
render() {
return (
<View style={{alignItems: 'center'}}>
<ColorPicker
ref={view => {this.colorPicker = view;}}
oldColor={this.state.oldColor}
onColorChange={this.changeColor}
textSaturation={'Saturation'}
diamond={true}
staticPalette={true}/>
</View>
);
}
}
Props
Prop | Type | Optional | Default | Description |
---|---|---|---|---|
oldColor | Color string | Yes | undefined | Initial positon of the picker indicator |
onColorChange | function | Yes | Callback called while the user click a color or release the slider. The 1st argument is color in HSV representation (see below). The 2nd string argument is always 'end'. | |
hideSliders | bool | Yes | false | Set this to true to hide the saturation sliders. |
textSaturation | string | Yes | 'Saturation' | Set the title text of the saturation slider. |
diamond | bool | Yes | true | Show diamond or rectangle palette. |
staticPalette | bool | Yes | true | Set this to false to let the slider change the saturation of palette. |
HSV color representation is an object literal with properties:
{
h: number, // <0, 360>
s: number, // <0, 1>
v: number, // <0, 1>
}
Donate
To support my work, please consider donate.
ETH: 0xd02fa2738dcbba988904b5a9ef123f7a957dbb3e