README
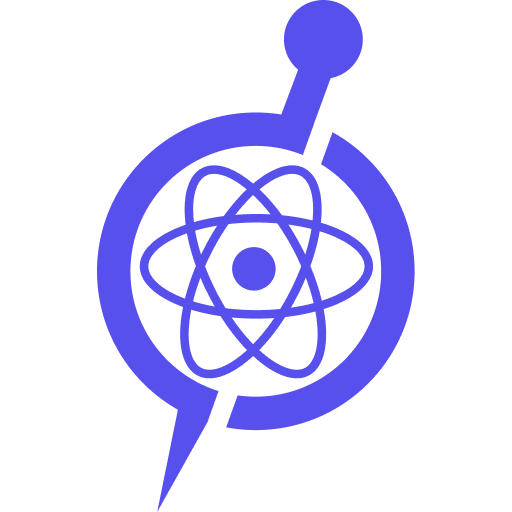
React Pinpoint
An open source utility library for measuring React component render times.
Table of Contents
Prerequisites
Browser context
React pinpoint must run inside a browser context to observe the React fiber tree. We recommended using automation software such as puppeteer to achieve this.
Production build with a twist
React optimises your development code base when you build for production, which decreases component render times. Users should therefore run react pinpoint against their production code
However, tweaks need to be made to the build process to preserve component names and enable render time profiling. Steps for doing so can be found here.
APIs
record(page, url, rootId)
page
<Page> Puppeteeer page instanceurl
<string> address React page is hosted atrootId
<string> id of dom element that React is mounted to- returns: <Page> Puppeteeer page instance with a listener attached to React components
This function attaches a listener to the Puppeteer page's react root for recording changes
report(page, threshold)
page
<Page> Puppeteeer page instance with record listener attachedthreshold
<Number> cutoff for acceptable component render time (in ms)- default time is 16ms
- returns: Node[] An array of nodes belonging to each react component that exceeded the given render time threshold
Will report all component render times that exceed the given threshold in ms
If no threshold is given, the default threshold of 16ms is used (please see FAQ “Why is the default render threshold 16ms?”)
Examples
Using with Puppeteer
const puppeteer = require('puppeteer');
const reactPinpoint = require('react-pinpoint');
(async () => {
const browser = await puppeteer.launch({});
const page = await browser.newPage();
// Pass information to
const url = 'http://localhost:3000/calculator';
const rootId = '#root';
await reactPinpoint.record(page, url, rootId);
// Perform browser actions
await page.click('#yeah1');
await page.click('#yeah2');
await page.click('#yeah3');
// Get all components that took longer than 16ms to render during browser actions
const threshold = 16;
const slowRenders = await reactPinpoint.reportTestResults(page, threshold);
await browser.close();
})();
Getting Started
- Head over to the React Pinpoint website.
- Register for an account.
- Add a project and fill in the details.
- Copy the project ID provided.
Installation
Using npm:
npm install -D react-pinpoint
Using yarn:
yarn add react-pinpoint -D
- Invoke
mountToReactRoot
and paste the project ID you received from the website as the second argument in your React project’s entry file:
mountToReactRoot(rootDom, projectID);
- Interact with your app and data will be sent to React Pinpoint website.
- Refresh the page and see your data displayed!
Docker
React pinpoint was designed with the goal of regression testing component render times within a CICD, we therefore offer several preconfigured docker containers to assist with using React pinpoint within a CICD as well as with examples for doing so.
FAQs
Why does React Pinpoint only measure individual component render times?
Since React has moved to using a React Fiber infrastructure, different component types are assumed to generate different trees.
Why is the default render threshold 16ms?
The recommended render time is 60 FPS.
Does React pinpoint work in a headless browser?
Yes! Due to the component render times being driven by component logic, a GUI is not needed to capture them.